How to increment operators’ work in the c program
Which was developed in the C program language, and shows how to use the increment operators (‘++’) and the ‘printf’ function to print values. Here is a detailed breakdown:
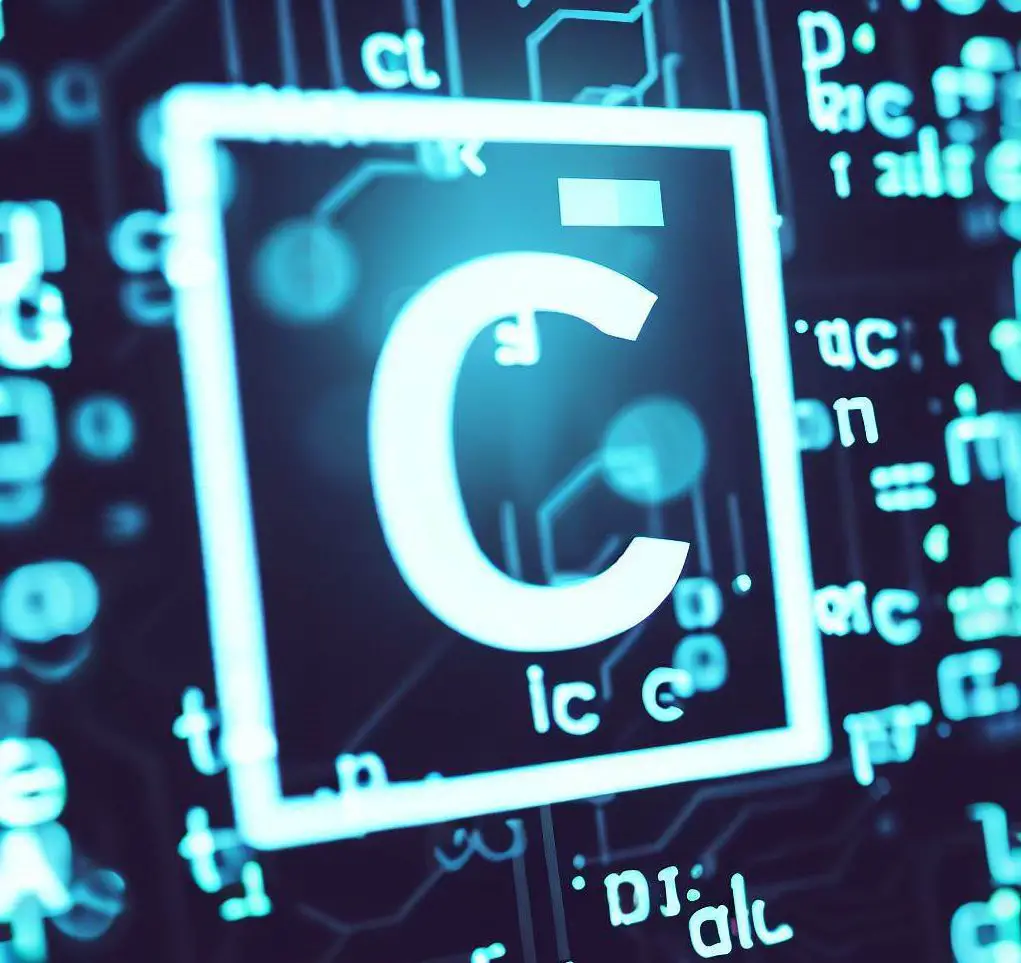
why do we use #include stdio.h in the c program?
We use the #include <stdio.h>
directive in a C program to include the standard input-output library, which provides functions for input and output operations.
#include <stdio.h>
void main()
{
int a = 7;
printf("%d", a++);
printf("\n%d", a++ * a++);
printf("\n%d", ++a * ++a);
}
Essential C functions like printf() and scanf() for producing output and reading input, respectively, are provided by the stdio.h library. We can utilise these functions in our C programme by using this library, which makes it simpler to communicate with the user and show information on the screen.
'#include <stdio.h>'
: This line includes the code’s standard input/output library, providing functions like'printf
‘ for printing output.'void main()
‘: This is the primary function where the program execution starts.- ‘
int a = 7;
‘: This line declares an integer variable'a
‘ and initializes it with the value 7. - ‘
printf("%d", a++);'
: This line prints the value of'a'
the console using the'printf
‘ function. The format specifier ‘%d
‘ indicates an integer value. The ‘a++'
operation is a post-increment operator, which means the value of ‘a'
is first used in the expression and then incremented by 1. Therefore, it prints'7
‘. 'printf("\n%d", a++ * a++);'
: This line also uses'printf
‘ to print a value. The expression'a++ * a++
‘ is evaluated from left to right. The post-increment operator ‘a++'
increments the valuea
by 1 after it has been used in the expression. In this case,'a
‘ is first multiplied by itself (‘7 * 8
‘), resulting in'56
‘. Then,'a
‘ is incremented twice, becoming'9
‘. Therefore, it prints'72
‘ to the console.'printf("\n%d", ++a * ++a);
‘: Similarly, this line uses'printf'
to print a value. The expression'++a * ++a'
is evaluated from left to right. The pre-increment operator ‘++a'
increments the valuea
by 1 before it is used in the expression. In this case,a
is incremented to'10
‘ and multiplied by itself ('10 * 11'
), resulting in'110
‘. The value of'a
‘ is incremented again, becoming'11'
. Therefore, it prints'132
‘ to the console.
The output of the code will be:
output:
7
72
132