level adjustment Program in C and Java
level adjustment Program in c
The level adjustment C programming language shows how to print out numbers and computations using variables, expressions, and printf instructions. I’ll explain the code and its results to you.
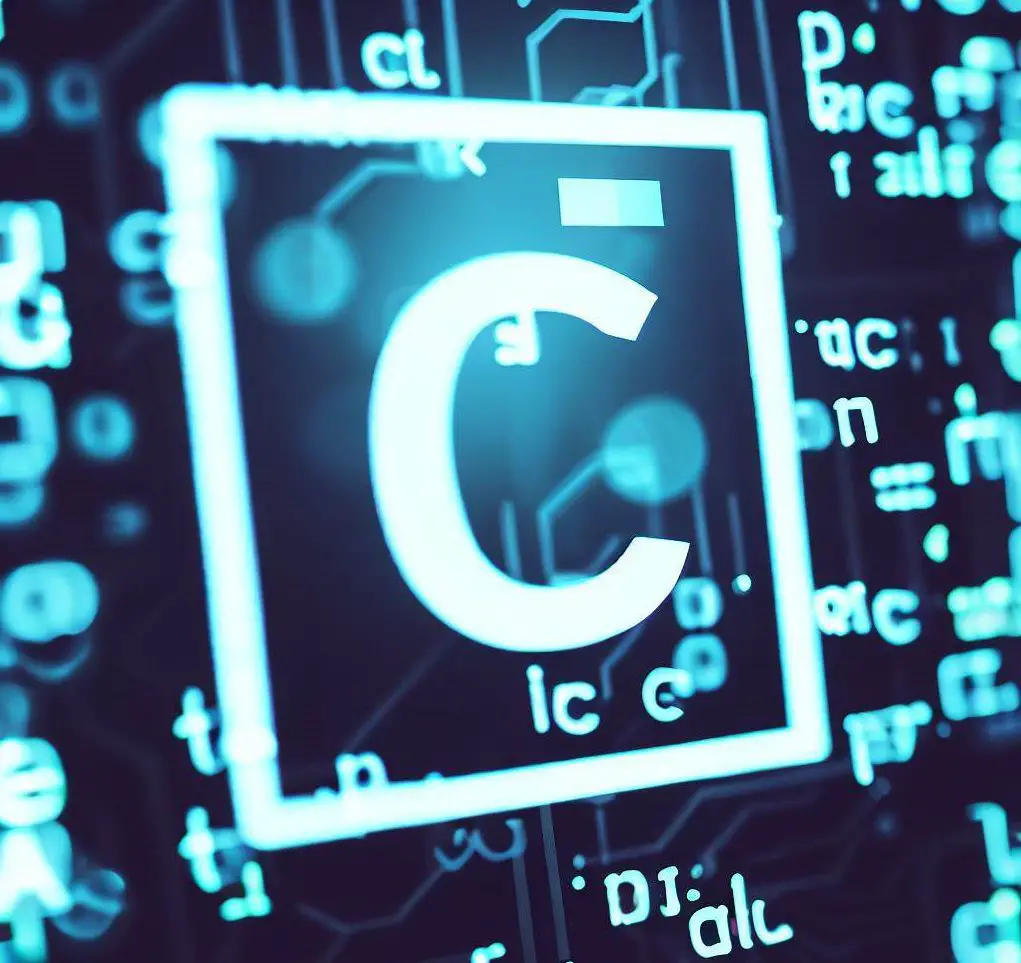
level adjustment Program in C
#include <stdio.h>
void main()
{
int x=420, y=777, z=1230, w=4440;
printf("%d/n%d/n%d/n%d", x, y, z, w);
printf("/t%d/n/t%d/n%d/n/t%d", x, y, z, w)
printf("%d%d", x+z-y*y, (y-z%w);
}
output:
420
777
1230
4440 420
777
1230
4440 -12255 6348
The standard input-output library is first included in the code by using #include<stdio.h>, which provides the essential functions for input and output operations.
The main function, void main(), is where the program execution starts.
The declaration and initialization of four integer variables (x, y, z, and w) are completed.
These variables’ values as well as the output of the expressions are printed using the printf instructions.
Using %d as the format specifier for integers and n to signify a new line, the first printf command outputs the values of x, y, z, and w on separate lines.
The first printf command outputs identical values as the second one, except each value is preceded by the tab character (t). The output is somewhat indented as a result.
The third printf articulation ascertains and prints the aftereffect of two articulations. The primary articulation is x + z – y * y, and the subsequent articulation is (y – z) % w. The % image is the modulo administrator, which ascertains the rest of the division.
level adjustment Program in Java
a common class Main: This line introduces the class Main. Every Java application needs a class, and in this case, the class name should match the filename (“Main.java”). The main method, the Java program’s entry point, is contained in this class.
(String[] args) public static void main This is the declaration of the main method. It is a unique Java method that acts as the program’s initial point of execution. It accepts a parameter named args, an array of strings that may be used to send command-line arguments to programs.
public class Main {
public static void main(String[] args) {
int x = 420, y = 777, z = 1230, w = 4440;
System.out.println(x);
System.out.println(y);
System.out.println(z);
System.out.println(w);
System.out.print("\t" + x + "\n\t" + y + "\n\t" + z + "\n\t" + w);
System.out.println(x + z - y * y);
System.out.println(y - z % w);
}
}
420
777
1230
4440
420
777
1230
4440
-12255
6348
These lines define and set the initial values of the four integer variables x, y, z, and w.
The values of the variables x, y, z, and w are printed to the console on different lines using the System.out.println function in these lines. This is comparable to printf in C, although it ends with a newline character by default.
The values of x, y, z, and w are printed in this line using System.out.print, with tabs (t) placed before each value and newlines (n) placed after each value. The values are written on the same line since it doesn’t automatically add a newline character at the end.
These lines compute and display the outcomes of the following two mathematical expressions:
x + z – y * y: It determines the outcome of x + z less y squared. The outcome is displayed on a single line.
y – z% w: It determines the outcome by dividing z by w and subtracting the remainder from y. On the next line, the outcome is displayed.
This Java application will create the variables, print their values, and calculate and print the answers to the two mathematical expressions as seen in the output when you execute it.