Largest numbers in c, java, python and flowchart
Table of Contents
Algorithm largest numbers
Draw the flowchart and write an algorithm to find the largest number of a given number.
The programme searches through a list of user-provided input numbers to identify the biggest one.
- Each input number is compared with the most recent highest number discovered so far using a loop.
- The computer programme anticipates that the user will submit true figures. Neither input validation nor error handling is done by it. The programme may generate unexpected results or crash if the user inputs non-numeric data.
- With the first input value, the programme initialises the greatest number. This guarantees that a baseline for comparison exists.
- By determining if the most recent input number exceeds the greatest number, a comparison is made. If so, the biggest number is changed to reflect the new value.
- Not all of the inputted numbers are kept in memory by the programme. Instead, it merely records the most significant number discovered thus far. This method conserves memory.
- The application anticipates at least one number to be entered by the user. If no numbers are entered, the greatest number’s starting value will remain the same, which will produce inaccurate results.
- The printf() method is used by the programme to show the user the greatest number.
- After showing the biggest number, the programme ends. You may surround the code in a loop or adapt the programme if you wish to carry out several iterations or let the user continually discover the biggest number.
- This is the algorithm to find the largest of three numbers a,b, and c – Accepted numbers
- To utilise the essential functions, it’s crucial to include the relevant header files at the start of the programme, such as stdio.h.
Algorithm largest numbers code
step 1 Start
step 2 [Accepted three numbers]
read a,b,c,
step 3 if a > b then
if a > c then
Print a is the largest
Else
Print c is the largest
Else
if b > c then
Print b is the largest
Else
Print c is the largest
step 4: stop
Flowchart largest numbers
With a flowchart, find the greatest number among a set of input numbers.
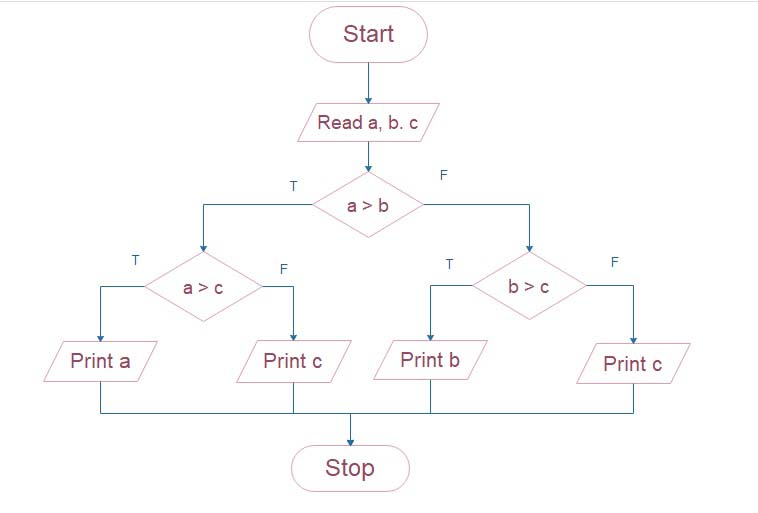
- Start: The “Start” sign marks the beginning of the program in the flowchart.
- Declare and initialize variables to hold both the input numbers and the greatest number thus far. For instance, you may set “num” and “largest” to 0.
- Total Elements to Input: Request that the user provide the total number of elements that they wish to compare. Put this amount in a variable and give it the name “total elements.
- Set Counter: Increase “counter,” a counter variable, to 1. This variable will be used to record the element that is being processed right now.
- Requesting the first number from the user, save it in the “num” variable.
- Compared to Largest: The value of “num” is compared to the largest number that is currently recorded in the “largest” variable.
- Update biggest: If “num” exceeds the value of “largest,” update “largest” so that it is equal to “num.”
- Increase Counter: To advance to the following element, increase the “counter” variable by 1.
- Verify Counter: Verify that the variable “counter” is either less than or equal to “totalElements.” If so, return to Step 5 and enter the following number before repeating the comparison procedure.
- Largest Output: If the “counter” variable exceeds “total elements,” then all items have been processed. The greatest number should be output as the “largest” value.
- Stop: The “Stop” sign at the flowchart’s conclusion denotes the conclusion of the program.
The steps of the flowchart are shown in the following text:
Start
|
Initialize Variables (num = 0, largest = 0)
|
Input Total Elements (totalElements)
|
Set Counter (counter = 1)
|
Input Number (num)
|
Compare with Largest (num vs. largest)
|
Update Largest (if num > largest, set largest = num)
|
Increment Counter (counter++)
|
Check Counter (counter <= totalElements?)
| |
| Yes
| |
| No
| |
| Output Largest (largest)
|
Stop
This flowchart shows how to use a loop and conditional expressions to discover the greatest number among the input integers. The greatest numbers program outlined previously will result from putting these procedures into practice in your selected programming language.
Largest number in C
How to the largest number of programs in c?
The total number of items (n) that the user wants to compare must be entered into this programme. The user is then prompted to enter each number individually. It uses a for loop to compare the greatest variable with each new number input after initialising it with the first number. The biggest value is updated when a value larger than the currently entered largest is input. It then shows the greatest number discovered.
program in C that allows you to find the largest number among a series of input numbers
#include <stdio.h>
int main() {
int n, i;
float num, largest;
printf("Enter the total number of elements: ");
scanf("%d", &n);
printf("Enter number 1: ");
scanf("%f", &largest);
for (i = 2; i <= n; i++) {
printf("Enter number %d: ", i);
scanf("%f", &num);
if (num > largest)
largest = num;
}
printf("The largest number is %.2f\n", largest);
return 0;
}
The software takes the input numbers to be floating-point numbers. Change the program’s data types from float to int if you wish to compare integers.
Largest numbers in Python
The largest number among a series of input numbers in Python:
def find_largest_number():
total_elements = int(input("Enter the total number of elements: "))
largest = float(input("Enter number 1: ")) # Initialize 'largest' with the first number
for i in range(2, total_elements + 1):
num = float(input(f"Enter number {i}: "))
if num > largest:
largest = num
print(f"The largest number is {largest}")
find_largest_number()
- The logic for determining the greatest number is included in the find_largest_number() function, which is defined.
- The user is asked how many components in total they wish to compare.
- The first input integer serves as the starting value for the variable biggest. To make sure we can handle floating-point integers, we utilize float().
- The for loop starts at 2 and extends up to total_elements + 1, as the first number has already been recorded individually.
- The user is requested to input each succeeding number inside the loop.
- If the input number is higher than the greatest number already in use, the software verifies this. If so, the new value is added to the largest’s value.
- Until all of the items have been handled, the loop continues.
- The biggest integer discovered by print() is shown by the application when the loop is finished.
- The reasoning is carried out by calling the find_largest_number() method at the conclusion of the program.
Now, if you execute this Python program, it will prompt you to input each number individually after asking you for the total number of components you wish to compare. The greatest number among the input numbers you supplied will then be shown.
Largest numbers in Java
How to find the largest number among a series of input numbers in Java:
import java.util.Scanner;
public class FindLargestNumber {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter the total number of elements: ");
int totalElements = input.nextInt();
System.out.print("Enter number 1: ");
double largest = input.nextDouble(); // Initialize 'largest' with the first number
for (int i = 2; i <= totalElements; i++) {
System.out.print("Enter number " + i + ": ");
double num = input.nextDouble();
if (num > largest) {
largest = num;
}
}
System.out.println("The largest number is " + largest);
input.close();
}
}
- The Scanner class from the java.util package is first imported by the Java application. The Scanner class is utilized to read user input.
- To read user input, we construct a new Scanner object named input in the main() function.
- The total number of components that the user wants to compare using the System must be entered.both input.nextInt() and out.print().
- The first input number is used to initialize the variable greatest utilizing input.nextDouble(). In order to handle floating-point values, we need the double data type.
- The program uses a for loop that goes from 2 to total elements. The loop will repeat in order to record each new number.
- The user is requested to input each number using the System during the loop.out.input and print().nextDouble().
- If the input number is higher than the greatest number already in use, the software verifies this. If so, an if statement is used to update the value of the biggest with the new value.
- Until all of the items have been handled, the loop continues.
- The software uses System.out.println() to find the greatest integer and shows it once the loop is finished.
- At the conclusion of the program, the Scanner object is closed using input.close() to relinquish the resources connected to it.
Now, if you execute this Java application, it will prompt you to enter each number individually after asking you to input the total number of items you wish to compare. The greatest number among the input numbers you supplied will then be shown.