Fibonacci sequence in c, python, java and Flowchart
Fibonacci sequence
Write an algorithm and draw the flowchart to display the Fibonacci series for n terms
Table of Contents
what is Fibonacci series
Each number in the Fibonacci series, which often begins with 0 and 1, is equal to the sum of the two numbers before it. The following is the order of events.
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, and so on…
Starting with the initial two integers (0 and 1), you may construct the Fibonacci series by adding the two numbers before it. Examples include 1 (the third number), which is the sum of 0 and 1, 2, which is the sum of 1 and 1, 3, which is the sum of 1 and 2, and so on.
This sequence regularly occurs in nature, the arts, and numerous scientific disciplines, and it has many intriguing mathematical features. The golden ratio, or around 1.61803398875, is a measure of proportion. The ratio of successive Fibonacci numbers is close to this value. Leonardo of Pisa, often known as Fibonacci, an Italian mathematician, popularized the series in his work “Liber Abaci” in 1202, which gave it its name.
Algorithm Fibonacci sequence
- This is the flowchart to display the Fibonacci series for n terms
- n-Accepted number
- i-loop variable
- f1,f2,f3,- variable to manage Fibonacci series
0 and 1 should be used as the initial two integers to create the Fibonacci sequence. Then, repeat step 2 through whichever many sequences you like. Add the previous two numbers to determine the next number at each stage.
Update the two preceding numbers and keep the new number as the most recent. The Fibonacci sequence will occur from continuing the loop until it reaches the appropriate length.
As an illustration, start with 0, 1, then 0 + 1 = 1, 1 + 1 = 2, 1 + 2 = 3, 2 + 3 = 5, and so on, until the sequence has the necessary length.
Algorithm Fibonacci series steps
step 1: Start
step 2: [Accept a number of terms] Read n
step 3: set i=1, f2=0,f3=1,
step 4: Repeat steps 5,6 and7until i<=n
step 5: [Display terms in series] Print f3
step 6: set f1=f2, f2=f3, f3=f1+f3
step 7: seti=i+1
step 8: Stop
Flowchart Fibonacci sequence
A flowchart showing the procedures used to create the Fibonacci series
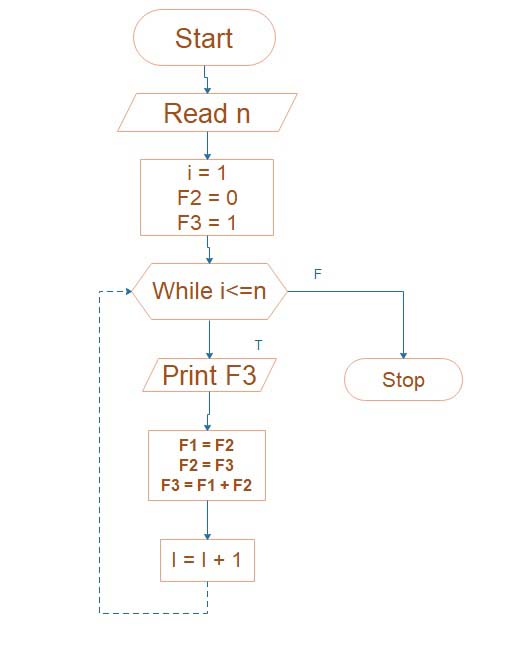
Flowchart Fibonacci series steps
The “Start” icon marks the beginning of the flowchart.
The variables a, b, and count are initialized with the values a = 0 for the first number, b = 1 for the second, and count = 2 for the reason that there are already two numbers in the series.
The required sequence length is input, which is then saved in the variable limit.
Conditional statements (IF) are used in the flowchart to handle unusual cases:
- If the limit is less than or equal to 0, an error message is printed before the program finishes.
- If the limit is 1, the Fibonacci sequence’s initial integer (zero) is printed before stopping.
- It publishes the first two integers in the series (0 and 1) if the limit is 2 or more.
Start
|
V
Initialize variables: a=0, b=1, count=2, limit (desired sequence length)
|
V
IF limit <= 0
| |
| V
| Print "Invalid input. The sequence length must be greater than zero."
| |
| V
| Stop
|
V
IF limit == 1
| |
| V
| Print "Fibonacci Sequence:", a
| |
| V
| Stop
|
V
IF limit >= 2
| |
| V
| Print "Fibonacci Sequence:", a, b
| |
| V
| WHILE count < limit
| | |
| | V
| | Compute next Fibonacci number: c = a + b
| | |
| | V
| | Print c
| | |
| | V
| | Update variables: a = b, b = c, count = count + 1
| |
| V
| End WHILE
|
V
Stop
The following Fibonacci numbers are calculated and printed in a loop (WHILE) until the required length of the sequence is attained if the limit is greater than or equal to 3.
The following Fibonacci number is determined within the loop by adding the previous two figures (a and b), and it is then saved in the variable c.
We display the most recent Fibonacci number (c).
By changing the values of the variables, we may advance to the following point in the sequence: a becomes the old value of b, b becomes the old value of c, and the count variable is increased by 1.
Until the count exceeds the limit, the loop continues. At that moment, the flowchart terminates.
Fibonacci sequence in c
The Fibonacci sequence implemented in C
#include <stdio.h>
int main() {
int limit, a = 0, b = 1, c;
printf("Enter the number of terms for the Fibonacci sequence: ");
scanf("%d", &limit);
if (limit <= 0) {
printf("Invalid input. The number of terms must be greater than zero.\n");
} else if (limit == 1) {
printf("Fibonacci Sequence: %d\n", a);
} else if (limit == 2) {
printf("Fibonacci Sequence: %d, %d\n", a, b);
} else {
printf("Fibonacci Sequence: %d, %d, ", a, b);
for (int count = 2; count < limit; count++) {
c = a + b;
printf("%d, ", c);
a = b;
b = c;
}
printf("\n");
}
return 0;
}
Fibonacci series in c
The user is prompted by this C software to specify the number of phrases they desire to be included in the Fibonacci sequence. The Fibonacci sequence is then generated and printed up to the specified limit.
When the limit is less than or equal to 0, 1, or 2, the software also handles those situations independently. Beyond the first two (0 and 1), the remaining Fibonacci numbers are calculated and printed using the for loop.
Fibonacci sequence in c++
The Fibonacci sequence implemented in C++
#include <iostream>
int main() {
int limit, a = 0, b = 1, c;
std::cout << "Enter the number of terms for the Fibonacci sequence: ";
std::cin >> limit;
if (limit <= 0) {
std::cout << "Invalid input. The number of terms must be greater than zero." << std::endl;
} else if (limit == 1) {
std::cout << "Fibonacci Sequence: " << a << std::endl;
} else if (limit == 2) {
std::cout << "Fibonacci Sequence: " << a << ", " << b << std::endl;
} else {
std::cout << "Fibonacci Sequence: " << a << ", " << b << ", ";
for (int count = 2; count < limit; count++) {
c = a + b;
std::cout << c;
if (count < limit - 1) {
std::cout << ", ";
}
a = b;
b = c;
}
std::cout << std::endl;
}
return 0;
}
Fibonacci series in c++
This C++ application performs similarly to the C version that was previously released. The Fibonacci sequence is generated and printed up to the specified limit when the user is prompted to select the number of words they desire in it.
When the limit is less than or equal to 0, 1, or 2, the software also handles those situations independently. Beyond the first two (0 and 1), the remaining Fibonacci numbers are calculated and printed using the for loop. For user input and output operations, the software makes use of the C++ input/output stream (std::cin and std::cout).
Fibonacci sequence in Python
The Fibonacci sequence implemented in Python
def fibonacci_sequence(limit):
a, b = 0, 1
fibonacci_list = [a, b]
if limit <= 0:
print("Invalid input. The number of terms must be greater than zero.")
elif limit == 1:
print("Fibonacci Sequence:", a)
else:
print("Fibonacci Sequence:", a, ",", b, end=", ")
for _ in range(2, limit):
c = a + b
fibonacci_list.append(c)
print(c, end=", ")
a, b = b, c
print()
return fibonacci_list
# Example usage:
limit = int(input("Enter the number of terms for the Fibonacci sequence: "))
fibonacci_sequence(limit)
Fibonacci series in Python
The Fibonacci sequence function, which we construct in this Python code, generates the Fibonacci sequence up to the specified limit by taking the limit as an argument. The Fibonacci numbers are stored in the method using a list called fibonacci_list. When the limit is less than or equal to 0, 1, or 2, it handles each situation independently.
A last example of how to use the software asks the user how many terms they want in the Fibonacci sequence before calling the Fibonacci sequence function to construct and output the Fibonacci sequence.
Fibonacci sequence in Java
The Fibonacci sequence implemented in Java
import java.util.Scanner;
public class FibonacciSequence {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the number of terms for the Fibonacci sequence: ");
int limit = scanner.nextInt();
if (limit <= 0) {
System.out.println("Invalid input. The number of terms must be greater than zero.");
} else {
System.out.print("Fibonacci Sequence: ");
int a = 0, b = 1;
System.out.print(a + ", " + b);
for (int count = 2; count < limit; count++) {
int c = a + b;
System.out.print(", " + c);
a = b;
b = c;
}
System.out.println();
}
scanner.close();
}
}
Fibonacci series in Java
The Fibonacci sequence class, which we construct in this Java code, asks the user to select the maximum number of words they wish to appear in the Fibonacci sequence. The Fibonacci sequence is then generated and printed up to the specified limit by the application. When the limit is less than or equal to 0, 1, or 2, the software also handles those situations independently.
The software used a for loop to compute and output the Fibonacci numbers after the first two (0 and 1) and the Scanner class to read user input. The Fibonacci sequence is calculated and stored using the variables a, b, and c.